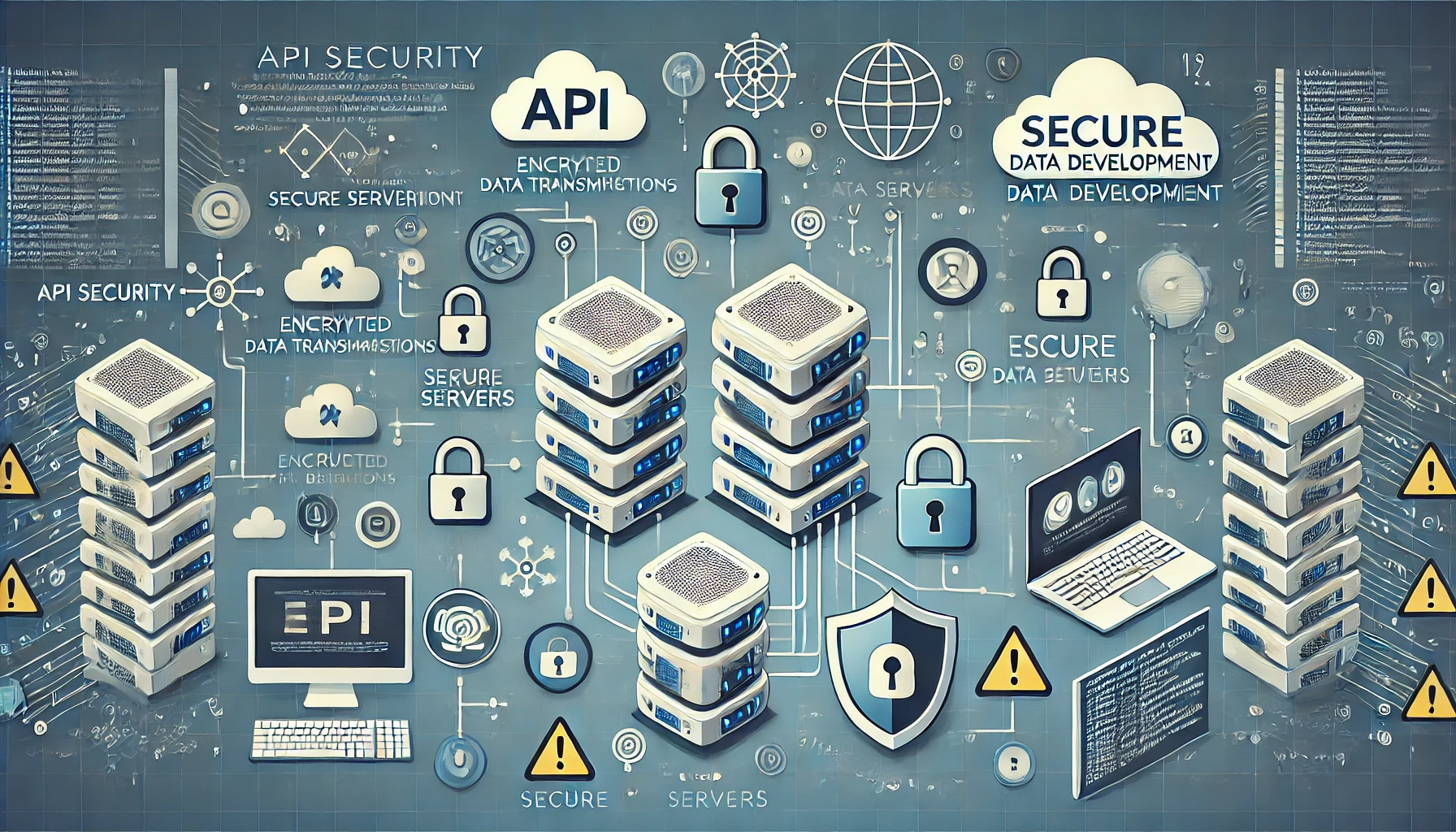
| Author: Abdullah Ahmed | Category: API Development and Integration
Best Practices for Secure API Development
In today's interconnected digital landscape, Application Programming Interfaces (APIs) play a vital role in facilitating communication between systems, enabling third-party integrations, and enhancing the functionality of applications. However, as APIs become more pervasive, they also become prime targets for malicious actors. Securing APIs is no longer an afterthought; it is a foundational element of the development process. In this blog, we will explore the best practices for secure API development, drawing on industry standards, real-world examples, and notable security breaches to illustrate why API security is crucial.
Understanding API Security
APIs allow different software systems to communicate and exchange data, making them indispensable in the development of modern applications. However, due to their openness, APIs can become vulnerable entry points for attackers if not properly secured. Some of the most common API security risks include:
- Broken Authentication: Weak authentication mechanisms allow unauthorized users to access sensitive data.
- Excessive Data Exposure: APIs may expose more data than necessary, giving attackers more information than they should have.
- Injection Attacks: APIs can be vulnerable to injection attacks (like SQL injection) if inputs are not properly validated.
- Rate Limiting and Denial of Service (DoS): If rate limiting is not enforced, APIs can be overwhelmed with requests, leading to service disruptions.
Now, let’s dive into industry best practices to secure APIs against such vulnerabilities.
1. Use HTTPS Everywhere
One of the foundational security practices in API development is using HTTPS instead of HTTP. HTTPS ensures that all data exchanged between the client and the server is encrypted, protecting it from interception by attackers. Without HTTPS, sensitive data such as authentication tokens, user credentials, and personal information can be easily exposed in transit.
Real-World Example
In 2014, Uber faced a major API vulnerability when security researchers discovered that their API was using HTTP instead of HTTPS for certain requests. This oversight allowed attackers to intercept sensitive data, exposing user information. Switching to HTTPS closed this security gap and improved their overall API security posture.
Best Practice
- Always use HTTPS to encrypt communication between clients and APIs.
- Ensure that SSL/TLS certificates are valid and updated regularly.
2. Implement Strong Authentication and Authorization
Authentication and authorization are critical components of API security. Authentication verifies the identity of the client making the API call, while authorization determines what the authenticated client is allowed to do.
Common Techniques for API Authentication
- OAuth 2.0: A widely used standard for token-based authentication. OAuth 2.0 provides temporary access tokens that limit the exposure of user credentials.
- API Keys: These are simple tokens passed along with the API request. Although effective for identifying the client, they are not suitable for user authentication.
- JWT (JSON Web Tokens): JWTs are used to securely transmit information between parties as a JSON object, providing a stateless and scalable authentication solution.
Real-World Example
In 2019, the Facebook-Cambridge Analytica scandal highlighted the dangers of improper API authentication and authorization. Third-party developers had access to far more user data than necessary due to weak API security policies. Had Facebook implemented stricter OAuth 2.0 permissions and better authorization mechanisms, they could have minimized the risk of data abuse.
Best Practice
- Use OAuth 2.0 for token-based authentication and authorization.
- Always validate user permissions before granting access to sensitive resources.
- Avoid using hardcoded API keys or credentials.
3. Secure API Endpoints with Rate Limiting
Rate limiting restricts the number of requests a client can make to an API within a given time frame. This prevents DoS attacks, where attackers overload the API with requests, rendering it unusable for legitimate users.
Real-World Example
In 2017, GitHub experienced a massive Distributed Denial of Service (DDoS) attack that flooded its API with an overwhelming number of requests. However, thanks to well-implemented rate limiting and DDoS protection strategies, GitHub was able to mitigate the attack with minimal downtime.
Best Practice
- Implement rate limiting and throttling to prevent abuse of your API.
- Ensure that clients are notified when rate limits are reached and provide guidance on how to resolve the issue.
4. Validate All Inputs
Unvalidated input is a common attack vector, particularly for injection attacks such as SQL injection and cross-site scripting (XSS). APIs must validate all inputs to ensure they are in the expected format and within acceptable ranges.
Real-World Example
In 2018, T-Mobile suffered a data breach due to a vulnerability in one of their APIs that allowed for unauthorized access to customer data. The breach occurred because the API did not properly validate the input, which allowed attackers to inject malicious queries and extract sensitive information from their systems.
Best Practice
- Always validate and sanitize input to prevent injection attacks.
- Use libraries or frameworks that provide built-in protection against common vulnerabilities such as SQL injection and XSS.
- Avoid trusting client-side validation and enforce validation on the server side.
5. Implement OAuth Scopes and Permissions
OAuth 2.0 scopes allow you to define the level of access that is granted to an application when it interacts with an API. By limiting access to specific functions or data, you reduce the potential for abuse or unauthorized access.
Real-World Example
Google APIs use OAuth 2.0 scopes extensively to control the level of access that third-party applications can have to user accounts. For example, a third-party app requesting access to a user’s Google Drive will only be granted permission for specific actions (e.g., view files) rather than full access.
Best Practice
- Use OAuth scopes to grant only the minimum required permissions for each application or user.
- Regularly review and update scopes as the API evolves.
6. Use IP Whitelisting and Geo-Fencing
IP whitelisting allows API access only from specific IP addresses, further securing access to sensitive API endpoints. Similarly, geo-fencing restricts access based on geographic location, which can be useful in limiting exposure to certain regions.
Real-World Example
Many financial institutions, such as Stripe, use IP whitelisting to ensure that API requests only come from trusted sources, reducing the risk of unauthorized access. Additionally, geo-fencing helps these institutions restrict API access to regions where they operate, lowering exposure to potential threats from outside their operational area.
Best Practice
- Use IP whitelisting for APIs that are designed to be consumed by specific clients or applications.
- Implement geofencing to restrict access from high-risk geographic locations.
7. Monitor and Log API Activity
API activity should be continuously monitored and logged for auditing and troubleshooting purposes. Logs help identify abnormal behavior, such as brute-force attacks or unexpected requests, and allow you to respond quickly to security incidents.
Real-World Example
In 2020, Twitter discovered a security breach in its API that allowed attackers to access account data by exploiting a bug. The issue was detected through API activity monitoring, and Twitter was able to quickly shut down the attack and notify affected users.
Best Practice
- Monitor all API activity, including successful and failed requests, to detect potential attacks.
- Implement robust logging practices and store logs in a secure, tamper-evident environment.
- Set up alerts for unusual API behavior, such as unexpected spikes in traffic or repeated failed login attempts.
8. Regularly Test and Audit Your APIs
API security is an ongoing process that requires regular testing, auditing, and updating. This includes conducting penetration testing, vulnerability assessments, and security audits to identify potential weaknesses in your APIs.
Real-World Example
In 2017, Equifax suffered one of the most significant data breaches in history, exposing the personal data of over 147 million people. The root cause was a vulnerability in an API endpoint that had not been properly tested or patched. Regular security audits could have detected and fixed this issue before it was exploited.
Best Practice
- Conduct regular security audits and penetration testing to identify potential vulnerabilities in your APIs.
- Keep your API libraries and dependencies up to date to minimize exposure to known vulnerabilities.
9. Use Token Expiry and Refresh Mechanisms
API tokens should have a short lifespan and be refreshed regularly to minimize the risk of token theft or misuse. Once a token expires, the user must authenticate again to obtain a new one, ensuring that compromised tokens cannot be used indefinitely.
Real-World Example
Slack and other popular APIs implement token expiry and refresh mechanisms to ensure that access tokens are valid only for a limited time. This prevents attackers from using stolen tokens to maintain unauthorized access to the API over long periods.
Best Practice
- Set token expiration times to limit the duration of access.
- Use token refresh mechanisms to provide seamless user experiences while maintaining security.
10. Ensure Backward Compatibility and Graceful Deprecation
When you release new API versions, make sure they are backward compatible with older versions to avoid breaking client applications. Gracefully deprecating outdated APIs gives clients time to transition without disrupting service.
Real-World Example
Stripe handles API versioning and deprecation gracefully by maintaining backward compatibility for existing API integrations and providing extensive documentation for migration. This allows developers to update their applications at their own pace without causing service disruptions.
Best Practice
- Maintain backward compatibility between API versions to avoid breaking client integrations.
- Notify clients in advance of deprecations and provide clear documentation for migration.
Conclusion
Secure API development requires a combination of strong authentication, regular testing, vigilant monitoring, and adherence to industry best practices. By implementing these strategies, you can protect your APIs from common attack vectors, ensure the safety of sensitive data, and maintain the trust of your users. APIs are the backbone of modern applications, and their security is paramount.